Hey there, fellow engineers and tech enthusiasts! I’m excited to share one of my favorite strategies for modern software delivery: combining Docker and Jenkins to power up your CI/CD pipelines.
Throughout my career as a Senior DevOps Engineer and Docker Captain, I’ve found that these two tools can drastically streamline releases, reduce environment-related headaches, and give teams the confidence they need to ship faster.
In this post, I’ll walk you through what Docker and Jenkins are, why they pair perfectly, and how you can build and maintain efficient pipelines. My goal is to help you feel right at home when automating your workflows. Let’s dive in.
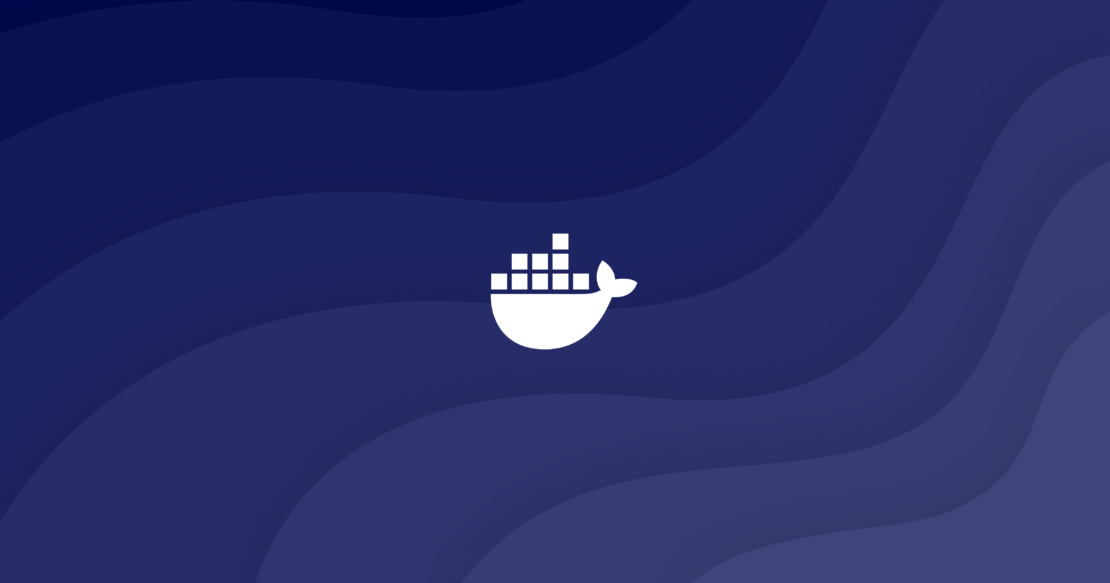
Brief overview of continuous integration and continuous delivery
Continuous integration (CI) and continuous delivery (CD) are key pillars of modern development. If you’re new to these concepts, here’s a quick rundown:
- Continuous integration (CI): Developers frequently commit their code to a shared repository, triggering automated builds and tests. This practice prevents conflicts and ensures defects are caught early.
- Continuous delivery (CD): With CI in place, organizations can then confidently automate releases. That means shorter release cycles, fewer surprises, and the ability to roll back changes quickly if needed.
Leveraging CI/CD can dramatically improve your team’s velocity and quality. Once you experience the benefits of dependable, streamlined pipelines, there’s no going back.
Why combine Docker and Jenkins for CI/CD?
Docker allows you to containerize your applications, creating consistent environments across development, testing, and production. Jenkins, on the other hand, helps you automate tasks such as building, testing, and deploying your code. I like to think of Jenkins as the tireless “assembly line worker,” while Docker provides identical “containers” to ensure consistency throughout your project’s life cycle.
Here’s why blending these tools is so powerful:
- Consistent environments: Docker containers guarantee uniformity from a developer’s laptop all the way to production. This consistency reduces errors and eliminates the dreaded “works on my machine” excuse.
- Speedy deployments and rollbacks: Docker images are lightweight. You can ship or revert changes at the drop of a hat — perfect for short delivery process cycles where minimal downtime is crucial.
- Scalability: Need to run 1,000 tests in parallel or support multiple teams working on microservices? No problem. Spin up multiple Docker containers whenever you need more build agents, and let Jenkins orchestrate everything with Jenkins pipelines.
For a DevOps junkie like me, this synergy between Jenkins and Docker is a dream come true.
Setting up your CI/CD pipeline with Docker and Jenkins
Before you roll up your sleeves, let’s cover the essentials you’ll need:
- Docker Desktop (or a Docker server environment) installed and running. You can get Docker for various operating systems.
- Jenkins downloaded from Docker Hub or installed on your machine. These days, you’ll want
jenkins/jenkins:lts
(the long-term support image) rather than the deprecatedlibrary/jenkins
image. - Proper permissions for Docker commands and the ability to manage Docker images on your system.
- A GitHub or similar code repository where you can store your Jenkins pipeline configuration (optional, but recommended).
Pro tip: If you’re planning a production setup, consider a container orchestration platform like Kubernetes. This approach simplifies scaling Jenkins, updating Jenkins, and managing additional Docker servers for heavier workloads.
Building a robust CI/CD pipeline with Docker and Jenkins
After prepping your environment, it’s time to create your first Jenkins-Docker pipeline. Below, I’ll walk you through common steps for a typical pipeline — feel free to modify them to fit your stack.
1. Install necessary Jenkins plugins
Jenkins offers countless plugins, so let’s start with a few that make configuring Jenkins with Docker easier:
- Docker Pipeline Plugin
- Docker
- CloudBees Docker Build and Publish
How to install plugins:
- Open Manage Jenkins > Manage Plugins in Jenkins.
- Click the Available tab and search for the plugins listed above.
- Install them (and restart Jenkins if needed).
Code example (plugin installation via CLI):
# Install plugins using Jenkins CLI
java -jar jenkins-cli.jar -s http://<jenkins-server>:8080/ install-plugin docker-pipeline
java -jar jenkins-cli.jar -s http://<jenkins-server>:8080/ install-plugin docker
java -jar jenkins-cli.jar -s http://<jenkins-server>:8080/ install-plugin docker-build-publish
Pro tip (advanced approach): If you’re aiming for a fully infrastructure-as-code setup, consider using Jenkins configuration as code (JCasC). With JCasC, you can declare all your Jenkins settings — including plugins, credentials, and pipeline definitions — in a YAML file. This means your entire Jenkins configuration is version-controlled and reproducible, making it effortless to spin up fresh Jenkins instances or apply consistent settings across multiple environments. It’s especially handy for large teams looking to manage Jenkins at scale.
Reference:
2. Set up your Jenkins pipeline
In this step, you’ll define your pipeline. A Jenkins “pipeline” job uses a Jenkinsfile
(stored in your code repository) to specify the steps, stages, and environment requirements.
Example Jenkinsfile:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git branch: 'main', url: 'https://github.com/your-org/your-repo.git'
}
}
stage('Build') {
steps {
script {
dockerImage = docker.build("your-org/your-app:${env.BUILD_NUMBER}")
}
}
}
stage('Test') {
steps {
sh 'docker run --rm your-org/your-app:${env.BUILD_NUMBER} ./run-tests.sh'
}
}
stage('Push') {
steps {
script {
docker.withRegistry('https://index.docker.io/v1/', 'dockerhub-credentials') {
dockerImage.push()
}
}
}
}
}
}
Let’s look at what’s happening here:
- Checkout: Pulls your repository.
- Build: Creates a built docker image (
your-org/your-app
) with the build number as a tag. - Test: Runs your test suite inside a fresh container, ensuring Docker containers create consistent environments for every test run.
- Push: Pushes the image to your Docker registry (e.g., Docker Hub) if the tests pass.
Reference: Jenkins Pipeline Documentation.
3. Configure Jenkins for automated builds
Now that your pipeline is set up, you’ll want Jenkins to run it automatically:
- Webhook triggers: Configure your source control (e.g., GitHub) to send a webhook whenever code is pushed. Jenkins will kick off a build immediately.
- Poll SCM: Jenkins periodically checks your repo for new commits and starts a build if it detects changes.
Which trigger method should you choose?
- Webhook triggers are ideal if you want near real-time builds. As soon as you push to your repo, Jenkins is notified, and a new build starts almost instantly. This approach is typically more efficient, as Jenkins doesn’t have to continuously check your repository for updates. However, it requires that your source control system and network environment support webhooks.
- Poll SCM is useful if your environment can’t support incoming webhooks — for example, if you’re behind a corporate firewall or your repository isn’t configured for outbound hooks. In that case, Jenkins routinely checks for new commits on a schedule you define (e.g., every five minutes), which can add a small delay and extra overhead but may simplify setup in locked-down environments.
Personal experience: I love webhook triggers because they keep everything as close to real-time as possible. Polling works fine if webhooks aren’t feasible, but you’ll see a slight delay between code pushes and build starts. It can also generate extra network traffic if your polling interval is too frequent.
4. Build, test, and deploy with Docker containers
Here comes the fun part — automating the entire cycle from build to deploy:
- Build Docker image: After pulling the code, Jenkins calls
docker.build
to create a new image. - Run tests: Automated or automated acceptance testing runs inside a container spun up from that image, ensuring consistency.
- Push to registry: Assuming tests pass, Jenkins pushes the tagged image to your Docker registry — this could be Docker Hub or a private registry.
- Deploy: Optionally, Jenkins can then deploy the image to a remote server or a container orchestrator (Kubernetes, etc.).
This streamlined approach ensures every step — build, test, deploy — lives in one cohesive pipeline, preventing those “where’d that step go?” mysteries.
5. Optimize and maintain your pipeline
Once your pipeline is up and running, here are a few maintenance tips and enhancements to keep everything running smoothly:
- Clean up images: Routine cleanup of Docker images can reclaim space and reduce clutter.
- Security updates: Stay on top of updates for Docker, Jenkins, and any plugins. Applying patches promptly helps protect your CI/CD environment from vulnerabilities.
- Resource monitoring: Ensure Jenkins nodes have enough memory, CPU, and disk space for builds. Overworked nodes can slow down your pipeline and cause intermittent failures.
Pro tip: In large projects, consider separating your build agents from your Jenkins controller by running them in ephemeral Docker containers (also known as Jenkins agents). If an agent goes down or becomes stale, you can quickly spin up a fresh one — ensuring a clean, consistent environment for every build and reducing the load on your main Jenkins server.
Why use Declarative Pipelines for CI/CD?
Although Jenkins supports multiple pipeline syntaxes, Declarative Pipelines stand out for their clarity and resource-friendly design. Here’s why:
- Simplified, opinionated syntax: Everything is wrapped in a single
pipeline { ... }
block, which minimizes “scripting sprawl.” It’s perfect for teams who want a quick path to best practices without diving deeply into Groovy specifics. - Easier resource allocation: By specifying an
agent
at either the pipeline level or within each stage, you can offload heavyweight tasks (builds, tests) onto separate worker nodes or Docker containers. This approach helps prevent your main Jenkins controller from becoming overloaded. - Parallelization and matrix builds: If you need to run multiple test suites or support various OS/browser combinations, Declarative Pipelines make it straightforward to define parallel stages or set up a matrix build. This tactic is incredibly handy for microservices or large test suites requiring different environments in parallel.
- Built-in “escape hatch”: Need advanced Groovy features? Just drop into a
script
block. This lets you access Scripted Pipeline capabilities for niche cases, while still enjoying Declarative’s streamlined structure most of the time. - Cleaner parameterization: Want to let users pick which tests to run or which Docker image to use? The
parameters
directive makes your pipeline more flexible. A single Jenkinsfile can handle multiple scenarios — like unit vs. integration testing — without duplicating stages.
Declarative Pipeline examples
Below are sample pipelines to illustrate how declarative syntax can simplify resource allocation and keep your Jenkins controller healthy.
Example 1: Basic Declarative Pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
}
}
stage('Test') {
steps {
echo 'Testing...'
}
}
}
}
- Runs on any available Jenkins agent (worker).
- Uses two stages in a simple sequence.
Example 2: Stage-level agents for resource isolation
pipeline {
agent none // Avoid using a global agent at the pipeline level
stages {
stage('Build') {
agent { docker 'maven:3.9.3-eclipse-temurin-17' }
steps {
sh 'mvn clean package'
}
}
stage('Test') {
agent { docker 'openjdk:17-jdk' }
steps {
sh 'java -jar target/my-app-tests.jar'
}
}
}
}
- Each stage runs in its own container, preventing any single node from being overwhelmed.
agent none
at the top ensures no global agent is allocated unnecessarily.
Example 3: Parallelizing test stages
pipeline {
agent none
stages {
stage('Test') {
parallel {
stage('Unit Tests') {
agent { label 'linux-node' }
steps {
sh './run-unit-tests.sh'
}
}
stage('Integration Tests') {
agent { label 'linux-node' }
steps {
sh './run-integration-tests.sh'
}
}
}
}
}
}
- Splits tests into two parallel stages.
- Each stage can run on a different node or container, speeding up feedback loops.
Example 4: Parameterized pipeline
pipeline {
agent any
parameters {
choice(name: 'TEST_TYPE', choices: ['unit', 'integration', 'all'], description: 'Which test suite to run?')
}
stages {
stage('Build') {
steps {
echo 'Building...'
}
}
stage('Test') {
when {
expression { return params.TEST_TYPE == 'unit' || params.TEST_TYPE == 'all' }
}
steps {
echo 'Running unit tests...'
}
}
stage('Integration') {
when {
expression { return params.TEST_TYPE == 'integration' || params.TEST_TYPE == 'all' }
}
steps {
echo 'Running integration tests...'
}
}
}
}
- Lets you choose which tests to run (unit, integration, or both).
- Only executes relevant stages based on the chosen parameter, saving resources.
Example 5: Matrix builds
pipeline {
agent none
stages {
stage('Build and Test Matrix') {
matrix {
agent {
label "${PLATFORM}-docker"
}
axes {
axis {
name 'PLATFORM'
values 'linux', 'windows'
}
axis {
name 'BROWSER'
values 'chrome', 'firefox'
}
}
stages {
stage('Build') {
steps {
echo "Build on ${PLATFORM} with ${BROWSER}"
}
}
stage('Test') {
steps {
echo "Test on ${PLATFORM} with ${BROWSER}"
}
}
}
}
}
}
}
- Defines a matrix of PLATFORM x BROWSER, running each combination in parallel.
- Perfect for testing multiple OS/browser combinations without duplicating pipeline logic.
Additional resources:
- Jenkins Pipeline Syntax: Official reference for sections, directives, and advanced features like matrix, parallel, and post conditions.
- Jenkins Pipeline Steps Reference: Comprehensive list of steps you can call in your Jenkinsfile.
- Jenkins Configuration as Code Plugin (JCasC): Ideal for version-controlling your Jenkins configuration, including plugin installations and credentials.
Using Declarative Pipelines helps ensure your CI/CD setup is easier to maintain, scalable, and secure. By properly configuring agents — whether Docker-based or label-based — you can spread workloads across multiple worker nodes, minimize resource contention, and keep your Jenkins controller humming along happily.
Best practices for CI/CD with Docker and Jenkins
Ready to supercharge your setup? Here are a few tried-and-true habits I’ve cultivated:
- Leverage Docker’s layer caching: Optimize your Dockerfiles so stable (less frequently changing) layers appear early. This drastically reduces build times.
- Run tests in parallel: Jenkins can run multiple containers for different services or microservices, letting you test them side by side. Declarative Pipelines make it easy to define parallel stages, each on its own agent.
- Shift left on security: Integrate security checks early in the pipeline. Tools like Docker Scout let you scan images for vulnerabilities, while Jenkins plugins can enforce compliance policies. Don’t wait until production to discover issues.
- Optimize resource allocation: Properly configure CPU and memory limits for Jenkins and Docker containers to avoid resource hogging. If you’re scaling Jenkins, distribute builds across multiple worker nodes or ephemeral agents for maximum efficiency.
- Configuration management: Store Jenkins jobs, pipeline definitions, and plugin configurations in source control. Tools like Jenkins Configuration as Code simplify versioning and replicating your setup across multiple Docker servers.
With these strategies — plus a healthy dose of Declarative Pipelines — you’ll have a lean, high-octane CI/CD pipeline that’s easier to maintain and evolve.
Troubleshooting Docker and Jenkins Pipelines
Even the best systems hit a snag now and then. Here are a few hurdles I’ve seen (and conquered):
- Handling environment variability: Keep Docker and Jenkins versions synced across different nodes. If multiple Jenkins nodes are in play, standardize Docker versions to avoid random build failures.
- Troubleshooting build failures: Use
docker logs -f <container-id>
to see exactly what happened inside a container. Often, the logs reveal missing dependencies or misconfigured environment variables. - Networking challenges: If your containers need to talk to each other — especially across multiple hosts — make sure you configure Docker networks or an orchestration platform properly. Read Docker’s networking documentation for details, and check out the Jenkins diagnosing issues guide for more troubleshooting tips.
Conclusion
Pairing Docker and Jenkins offers a nimble, robust approach to CI/CD. Docker locks down consistent environments and lightning-fast rollouts, while Jenkins automates key tasks like building, testing, and pushing your changes to production. When these two are in harmony, you can expect shorter release cycles, fewer integration headaches, and more time to focus on developing awesome features.
A healthy pipeline also means your team can respond quickly to user feedback and confidently roll out updates — two crucial ingredients for any successful software project. And if you’re concerned about security, there are plenty of tools and best practices to keep your applications safe.
I hope this guide helps you build (and maintain) a high-octane CI/CD pipeline that your team will love. If you have questions or need a hand, feel free to reach out on the community forums, join the conversation on Slack, or open a ticket on GitHub issues. You’ll find plenty of fellow Docker and Jenkins enthusiasts who are happy to help.
Thanks for reading, and happy building!
Learn more
- Subscribe to the Docker Newsletter.
- Take a deeper dive in Docker’s official Jenkins integration documentation.
- Explore Docker Business for comprehensive CI/CD security at scale.
- Get the latest release of Docker Desktop.
- Have questions? The Docker community is here to help.
- New to Docker? Get started.