If you’re anything like me, you love crafting sleek and responsive user interfaces with React. But, setting up consistent development environments and ensuring smooth deployments can also get complicated. That’s where Docker can help save the day.
As a Senior DevOps Engineer and Docker Captain, I’ve navigated the seas of containerization and witnessed firsthand how Docker can revolutionize your workflow. In this guide, I’ll share how you can dockerize a React app to streamline your development process, eliminate those pesky “it works on my machine” problems, and impress your colleagues with seamless deployments.
Let’s dive into the world of Docker and React!
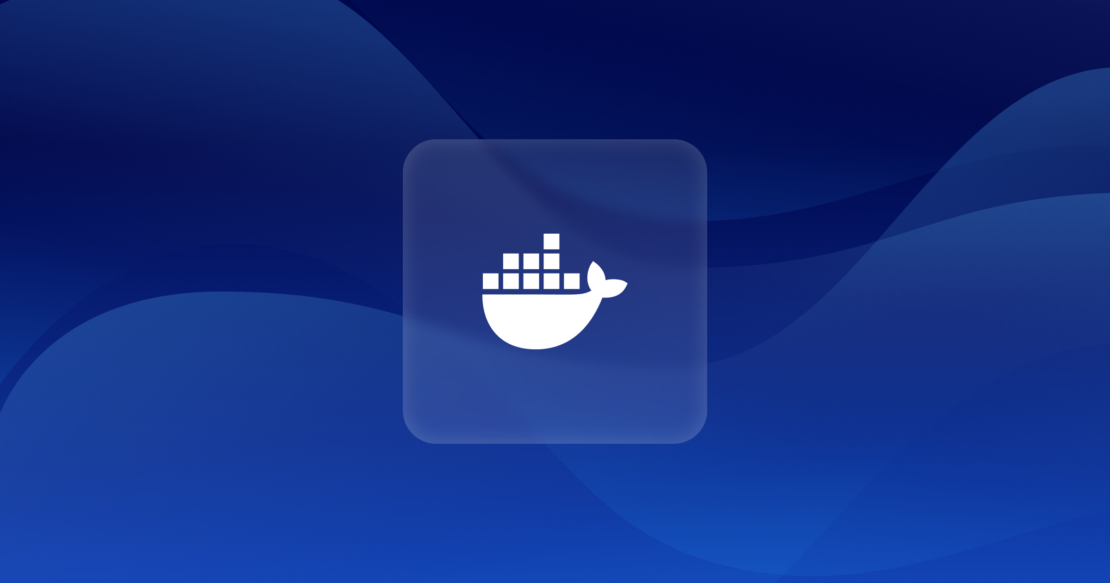
Why containerize your React application?
You might be wondering, “Why should I bother containerizing my React app?” Great question! Containerization offers several compelling benefits that can elevate your development and deployment game, such as:
- Streamlined CI/CD pipelines: By packaging your React app into a Docker container, you create a consistent environment from development to production. This consistency simplifies continuous integration and continuous deployment (CI/CD) pipelines, reducing the risk of environment-specific issues during builds and deployments.
- Simplified dependency management: Docker encapsulates all your app’s dependencies within the container. This means you won’t have to deal with the infamous “works on my machine” dilemma anymore. Every team member and deployment environment uses the same setup, ensuring smooth collaboration.
- Better resource management: Containers are lightweight and efficient. Unlike virtual machines, Docker containers share the host system’s kernel, which means you can run more containers on the same hardware. This efficiency is crucial when scaling applications or managing resources in a production environment.
- Isolated environment without conflict: Docker provides isolated environments for your applications. This isolation prevents conflicts between different projects’ dependencies or configurations on the same machine. You can run multiple applications, each with its own set of dependencies, without them stepping on each other’s toes.
Getting started with React and Docker
Before we go further, let’s make sure you have everything you need to start containerizing your React app.
Tools you’ll need
- Docker Desktop: Download and install it from the official Docker website.
- Node.js and npm: Grab them from the Node.js official site.
- React app: Use an existing project or create a new one using
create-react-app
.
A quick introduction to Docker
Docker offers a comprehensive suite of enterprise-ready tools, cloud services, trusted content, and a collaborative community that helps streamline workflows and maximize development efficiency. The Docker productivity platform allows developers to package applications into containers — standardized units that include everything the software needs to run. Containers ensure that your application runs the same, regardless of where it’s deployed.
How to dockerize your React project
Now let’s get down to business. We’ll go through the process step by step and, by the end, you’ll have your React app running inside a Docker container.
Step 1: Set up the React app
If you already have a React app, you can skip this step. If not, let’s create one:
npx create-react-app my-react-app
cd my-react-app
This command initializes a new React application in a directory called my-react-app
.
Step 2: Create a Dockerfile
In the root directory of your project, create a file named Dockerfile
(no extension). This file will contain instructions for building your Docker image.
Dockerfile for development
For development purposes, you can create a simple Dockerfile:
# Use the latest LTS version of Node.js
FROM node:18-alpine
# Set the working directory inside the container
WORKDIR /app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy the rest of your application files
COPY . .
# Expose the port your app runs on
EXPOSE 3000
# Define the command to run your app
CMD ["npm", "start"]
What’s happening here?
FROM node:18-alpine
: We’re using the latest LTS version of Node.js based on Alpine Linux.WORKDIR /app
: Sets the working directory inside the container.*COPY package.json ./**
: Copiespackage.json
andpackage-lock.json
to the working directory.RUN npm install
: Installs the dependencies specified inpackage.json
.COPY . .
: Copies all the files from your local directory into the container.EXPOSE 3000
: Exposes port 3000 on the container (React’s default port).CMD ["npm", "start"]
: Tells Docker to runnpm start
when the container launches.
Production Dockerfile with multi-stage build
For a production-ready image, we’ll use a multi-stage build to optimize the image size and enhance security.
# Build Stage
FROM node:18-alpine AS build
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production Stage
FROM nginx:stable-alpine AS production
COPY --from=build /app/build /usr/share/nginx/html
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
Explanation
- Build stage:
FROM node:18-alpine AS build:
Uses Node.js 18 for building the app.RUN npm run build
: Builds the optimized production files.
- Production stage:
FROM nginx
: Uses Nginx to serve static files.COPY --from=build /app/build /usr/share/nginx/html
: Copies the build output from the previous stage.EXPOSE 80
: Exposes port 80.CMD ["nginx", "-g", "daemon off;"]
: Runs Nginx in the foreground.
Benefits
- Smaller image size: The final image contains only the production build and Nginx.
- Enhanced security: Excludes development dependencies and Node.js runtime from the production image.
- Performance optimization: Nginx efficiently serves static files.
Step 3: Create a .dockerignore file
Just like .gitignore
helps Git ignore certain files, .dockerignore
tells Docker which files or directories to exclude when building the image. Create a .dockerignore
file in your project’s root directory:
node_modules
npm-debug.log
Dockerfile
.dockerignore
.git
.gitignore
.env
Excluding unnecessary files reduces the image size and speeds up the build process.
Step 4: Build and run your dockerized React app
Navigate to your project’s root directory and run:
docker build -t my-react-app .
This command tags the image with the name my-react-app
and specifies the build context (current directory). By default, this will build the final production stage from your multi-stage Dockerfile, resulting in a smaller, optimized image.
If you have multiple stages in your Dockerfile and need to target a specific build stage (such as the build
stage), you can use the --target
option. For example:
docker build -t my-react-app-dev --target build .
Note: Building with --target build
creates a larger image because it includes the build tools and dependencies needed to compile your React app. The production image (built using –target production
) on the other hand, is much smaller because it only contains the final build files.
Running the Docker container
For the development image:
docker run -p 3000:3000 my-react-app-dev
For the production image:
docker run -p 80:80 my-react-app
Accessing your application
Next, open your browser and go to:
http://localhost:3000
(for development)http://localhost
(for production)
You should see your React app running inside a Docker container.
Step 5: Use Docker Compose for multi-container setups
Here’s an example of how a React frontend app can be configured as a service using Docker Compose.
Create a compose.yml
file:
services:
web:
build: .
ports:
- "3000:3000"
volumes:
- .:/app
- ./node_modules:/app/node_modules
environment:
NODE_ENV: development
stdin_open: true
tty: true
command: npm start
Explanation
services:
Defines a list of services (containers).web:
The name of our service.build: .:
Builds the Dockerfile in the current directory.ports:
Maps port 3000 on the container to port 3000 on the host.volumes:
Mounts the current directory and node_modules for hot-reloading.environment:
Sets environment variables.stdin_open
andtty:
Keep the container running and interactive.
Step 6: Publish your image to Docker Hub
Sharing your Docker image allows others to run your app without setting up the environment themselves.
Log in to Docker Hub:
docker login
Enter your Docker Hub username and password when prompted.
Tag your image:
docker tag my-react-app your-dockerhub-username/my-react-app
Replace your-dockerhub-username
with your actual Docker Hub username.
Push the image:
docker push your-dockerhub-username/my-react-app
Your image is now available on Docker Hub for others to pull and run.
Pull and run the image:
docker pull your-dockerhub-username/my-react-app
docker run -p 80:80 your-dockerhub-username/my-react-app
Anyone can now run your app by pulling the image.
Handling environment variables securely
Managing environment variables securely is crucial to protect sensitive information like API keys and database credentials.
Using .env files
Create a .env
file in your project root:
REACT_APP_API_URL=https://api.example.com
Update your compose.yml
:
services:
web:
build: .
ports:
- "3000:3000"
volumes:
- .:/app
- ./node_modules:/app/node_modules
env_file:
- .env
stdin_open: true
tty: true
command: npm start
Security note: Ensure your .env
file is added to .gitignore
and .dockerignore
to prevent it from being committed to version control or included in your Docker image.
To start all services defined in a compose.yml in detached mode, the command is:
docker compose up -d
Passing environment variables at runtime
Alternatively, you can pass variables when running the container:
docker run -p 3000:3000 -e REACT_APP_API_URL=https://api.example.com my-react-app-dev
Using Docker Secrets (advanced)
For sensitive data in a production environment, consider using Docker Secrets to manage confidential information securely.
Production Dockerfile with multi-stage builds
When preparing your React app for production, multi-stage builds keep things lean and focused. They let you separate the build process from the final runtime environment, so you only ship what you need to serve your app. This not only reduces image size but also helps prevent unnecessary packages or development dependencies from sneaking into production.
The following is an example that goes one step further: We’ll create a dedicated build stage, a development environment stage, and a production stage. This approach ensures you can develop comfortably while still ending up with a streamlined, production-ready image.
# Stage 1: Build the React app
FROM node:18-alpine AS build
WORKDIR /app
# Leverage caching by installing dependencies first
COPY package.json package-lock.json ./
RUN npm install --frozen-lockfile
# Copy the rest of the application code and build for production
COPY . ./
RUN npm run build
# Stage 2: Development environment
FROM node:18-alpine AS development
WORKDIR /app
# Install dependencies again for development
COPY package.json package-lock.json ./
RUN npm install --frozen-lockfile
# Copy the full source code
COPY . ./
# Expose port for the development server
EXPOSE 3000
CMD ["npm", "start"]
# Stage 3: Production environment
FROM nginx:alpine AS production
# Copy the production build artifacts from the build stage
COPY --from=build /app/build /usr/share/nginx/html
# Expose the default NGINX port
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
What’s happening here?
- build stage: The first stage uses the official Node.js image to install dependencies, run the build, and produce an optimized, production-ready React build. By copying only your
package.json
andpackage-lock.json
before runningnpm install
, you leverage Docker’s layer caching, which speeds up rebuilds when your code changes but your dependencies don’t. - development stage: Need a local environment with hot-reloading for rapid iteration? This second stage sets up exactly that. It installs dependencies again (using the same caching trick) and starts the development server on port 3000, giving you the familiar
npm start
experience inside Docker. - production stage: Finally, the production stage uses a lightweight NGINX image to serve your static build artifacts. This stripped-down image doesn’t include Node.js or unnecessary development tools — just your optimized app and a robust web server. It keeps things clean, secure, and efficient.
This structured approach makes it a breeze to switch between development and production environments. You get fast feedback loops while coding, plus a slim, optimized final image ready for deployment. It’s a best-of-both-worlds solution that will streamline your React development workflow.
Troubleshooting common issues with Docker and React
Even with the best instructions, issues can arise. Here are common problems and how to fix them.
Issue: “Port 3000 is already in use”
Solution: Either stop the service using port 3000 or map your app to a different port when running the container.
docker run -p 4000:3000 my-react-app
Access your app at http://localhost:4000
.
Issue: Changes aren’t reflected during development
Solution: Use Docker volumes to enable hot-reloading. In your compose.yml
, ensure you have the following under volumes
:
volumes:
- .:/app
- ./node_modules:/app/node_modules
This setup allows your local changes to be mirrored inside the container.
Issue: Slow build times
Solution: Optimize your Dockerfile to leverage caching. Copy only package.json
and package-lock.json
before running npm install
. This way, Docker caches the layer unless these files change.
COPY package*.json ./
RUN npm install
COPY . .
Issue: Container exits immediately
Cause: The React development server may not keep the container running by default.
Solution: Ensure you’re running the container interactively:
docker run -it -p 3000:3000 my-react-app
Issue: File permission errors
Solution: Adjust file permissions or specify a user in the Dockerfile using the USER
directive.
# Add before CMD
USER node
Issue: Performance problems on macOS and Windows
File-sharing mechanisms between the host system and Docker containers introduce significant overhead on macOS and Windows, especially when working with large repositories or projects containing many files. Traditional methods like osxfs
and gRPC FUSE
often struggle to scale efficiently in these environments.
Solutions:
Enable synchronized file shares (Docker Desktop 4.27+): Docker Desktop 4.27+ introduces synchronized file shares, which significantly enhance bind mount performance by creating a high-performance, bidirectional cache of host files within the Docker Desktop VM.
Key benefits:
- Optimized for large projects: Handles monorepos or repositories with thousands of files efficiently.
- Performance improvement: Resolves bottlenecks seen with older file-sharing mechanisms.
- Real-time synchronization: Automatically syncs filesystem changes between the host and container in near real-time.
- Reduced file ownership conflicts: Minimizes issues with file permissions between host and container.
How to enable:
- Open Docker Desktop and go to Settings > Resources > File Sharing.
- In the Synchronized File Shares section, select the folder to share and click Initialize File Share.
- Use bind mounts in your
compose.yml
or Docker CLI commands that point to the shared directory.
Optimize with .syncignore
: Create a .syncignore
file in the root of your shared directory to exclude unnecessary files (e.g., node_modules, .git/
) for better performance.
Example .syncignore
file:
node_modules
.git/
*.log
Example in compose.yml
:
services:
web:
build: .
volumes:
- ./app:/app
ports:
- "3000:3000"
environment:
NODE_ENV: development
Leverage WSL 2 on Windows: For Windows users, Docker’s WSL 2 backend offers near-native Linux performance by running the Docker engine in a lightweight Linux VM.
How to enable WSL 2 backend:
- Ensure Windows 10 version 2004 or higher is installed.
- Install the Windows Subsystem for Linux 2.
- In Docker Desktop, go to Settings > General and enable Use the WSL 2 based engine.
Use updated caching options in volume mounts: Although legacy options like :cached
and :delegated
are deprecated, consistency modes still allow optimization:
consistent
: Strict consistency (default).cached
: Allows the host to cache contents.delegated
: Allows the container to cache contents.
Example volume configuration:
volumes:
- type: bind
source: ./app
target: /app
consistency: cached
Optimizing your React Docker setup
Let’s enhance our setup with some advanced techniques.
Reducing image size
Every megabyte counts, especially when deploying to cloud environments.
- Use smaller base images: Alpine-based images are significantly smaller.
- Clean up after installing dependencies:
RUN npm install && npm cache clean --force
- Avoid copying unnecessary files: Use
.dockerignore
effectively.
Leveraging Docker build cache
Ensure that you’re not invalidating the cache unnecessarily. Only copy files that are required for each build step.
Using Docker layers wisely
Each command in your Dockerfile creates a new layer. Combine commands where appropriate to reduce the number of layers.
RUN npm install && npm cache clean --force
Conclusion
Dockerizing your React app is a game-changer. It brings consistency, efficiency, and scalability to your development workflow. By containerizing your application, you eliminate environment discrepancies, streamline deployments, and make collaboration a breeze.
So, the next time you’re setting up a React project, give Docker a shot. It will make your life as a developer significantly easier. Welcome to the world of containerization!
Learn more
- Subscribe to the Docker Newsletter.
- Get the latest release of Docker Desktop.
- Have questions? The Docker community is here to help.
- New to Docker? Get started.